The Complete Python Split String Tutorial
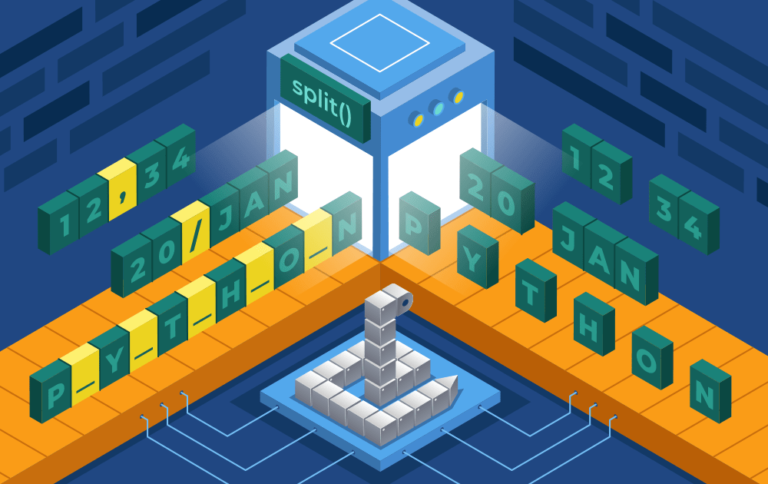
Strings are great tools for Python programmers. In Python, a string refers to a character sequence written inside quotes. String variables can have numeric/alphanumeric characters and they are mostly used to display/print messages or store directories. When using Python strings, you’ll need to split a string into an array of substrings using a particular delimiter. This is normally done using Python Split String.
In our tutorial, we’ll be discussing how to split Python strings. We’ll first discuss what Python’s Split function does its parameters. I’ll finally give practical examples that demonstrate how to use this function.
What does Split do in Python?
The split function scans through a string and then it separates it after encountering a separator.
The string must have one/more separating characters, which can be space.
The white space is the default separator.
This means if it doesn’t encounter the separator, it uses white space as a separator to split a string.
It functions this syntax:
string.split(separator,maxsplit)
The separator parameteris optional defining the separator to use to split a string.
The maxsplit parameter is also optional and it defines the total splits to perform. Its default value’s “-1” for all separator occurrences.
How to Split a String in Python
The .split() function separates the main string via a delimiter and returns a list of strings.
This python break string into list function returns the substrings in list data type.
Example:
my_st = ‘Sample Python string’
print(my_st.split())
In above example, we’ve declared the string my_st.
The string’s value was put inside single quotes.
We’ve then invoked .split() function to split it.
The example program returns this:

The output shows the string was split into substrings.
Another string splitting example:
my_st = ‘blue,orange,red’
print(my_st.split(“,”))
In above example, we’ve created string variable my_st with 3 strings.
The comma will act as a separator when splitting it.
The example program returns this:

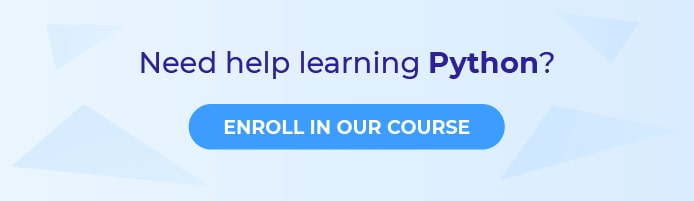
Different Ways of How to Split a String in Python
There are different ways to implement Python’s Split function.
They include:
Python Split String by Space
If no separator is given when calling.split() function, it’ll use whitespace to separate the string.
Example:
my_st = “Split Python String”
print(my_st.split())
The code returns:

We’ve invoked .split() function on string my_st.
Notice we didn’t specify the separator to use when calling .split() function, hence, it used white space as the separator.
Python Split String by Comma
You can use a comma (,) as the separator to split a string in Python.
It returns a list of strings contained between commas in your initial string.
Example of python split string into list by comma:
my_st = “This,is,a,Python,String”
print(my_st.split(“,”))
The code returns this output:

The string variable my_st was assigned values with commas (,) in between them.
We invoked .split() function on it and used comma as the separator.
The output is a list of substrings extracted from in-between commas in the original string.
Python String Split Multiple Delimiters
Multiple delimiters can be used to split a Python string.
You simply specify multiple characters as separators in the Split function.
A delimiter is one/more characters in a sequence used to mark bounds between regions in text.
Examples of delimiters are comma (,) and colon (:).
To split a string with multiple delimiters, use re.split() function.
Example:
import re
my_st = ‘I\nlove; Python*programming\nlanguage’
print(re.split(‘; |, |\*|\n’,my_st))
The code returns this output:

We’ve imported Python’s re module to use its regular expression functions and modules.
The variable my_st was declared and assigned a string with multiple delimiters including new line(\n), semi-colon(;), and asterisk(*).
The re.split() function was invoked on the string and delimiters specified as separators.
The output is a list of substrings that excludes the delimiters.
What does Split do in Python when used with maxsplit Parameter?
The maxsplit parameter helps us define the maximum number of splits the function should perform.
You can split your string by setting some value for this parameter.
For example, if whitespace is the separator and a maxsplit value of 1 is used, the string will be split into a list with a maximum of 2 items.
Example:
languages = “Python,Java,Perl, PHP,Swift”
print(languages.split(“,”,1))
We’ve declared variable languages with a string of different languages.
The split function takes a comma as the separator and 1 for maxsplit parameter.
This means the splitting will be done once, returning two lists of strings.
The code returns this output:

This example shows how to use split in python to perform a maximum of 2 splits on a string:
languages = “Python,Java,Perl, PHP,Swift”
print(languages.split(“,”,2))
In the above example, we’re using whitespace as the separator to perform a maximum of 2 splits on string languages.
The code returns this output:

Python Split a String in Half
The .split() function can’t split a string into two equal halves.
However, we can use slicing, a Python technique that involves combining the slice operator (:) with len() function to count the number of string characters.
Example:
languages = “Python,Java,Perl, PHP,Swift”
half1, half2 = languages[:len(languages)//2],languages[len(languages)//2:]
print(“First half : ” + half1)
print(“Second half : ” + half2)
The code returns this output:

The values of the string languages were split into equal halves. Notice we used the floor division operator (//)to work with whole integer values for length of string.
Conclusion:
This is what you’ve learned in this Python split string tutorial:
- The split function separates a string into substrings via a separator.
- You can use the maxsplit parameter to specify the maximum number of splits to do.
- If you don’t specify the type of separator to use, the function uses whitespace as its default separator.
- Use the slicing technique to split Python strings into equal halves.
To keep on getting more of such content, subscribe to our email newsletter now!
Also, don’t forget to share this article with others on the channels given below.
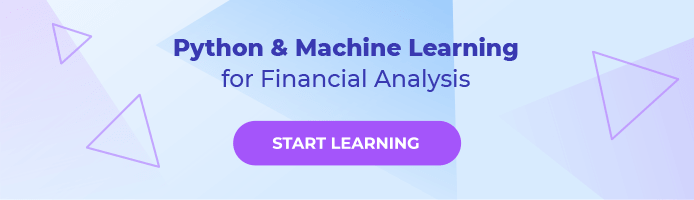